The SDK is flexible and does not impose any restrictions on what events you track in a presentation.
For example, if you have a multi-screen presentation, you probably want to record an event each time a user navigates from one screen to another. If your HTML has navigation links that look like this:
<ul>
<li><a href="products.html">Products</a></li>
<li><a href="product-a.html">Product A</a></li>
<li><a href="product-b.html">Product B</a></li>
<li><a href="about-us.html">About Us</a></li>
</ul>
Then you could use jQuery (or whichever framework you want) to log an event each time a user taps one of those links like this:
$('a').click(function() {
var target = $(this).attr('href');
//the first parameter is a category, the second is the action in that category.
//Both are arbitrary, but these are the recommended values for consistency
mobilelocker.logEvent('navigate', 'tap', target);
return false;
});
So if the user navigated from products
to product-a
, to about-us
, the following events will be logged:
mobilelocker.logEvent('navigate', 'tap', 'products.html');
mobilelocker.logEvent('navigate', 'tap', 'product-a.html');
mobilelocker.logEvent('navigate', 'tap', 'about-us.html');
The app will immediately log that event to the app's database. If you have an internet connection, it will also send it to the admin portal. To view the events it logs in each user session, go to Presentations > [ Your Presentation] > Sessions, and select the session.
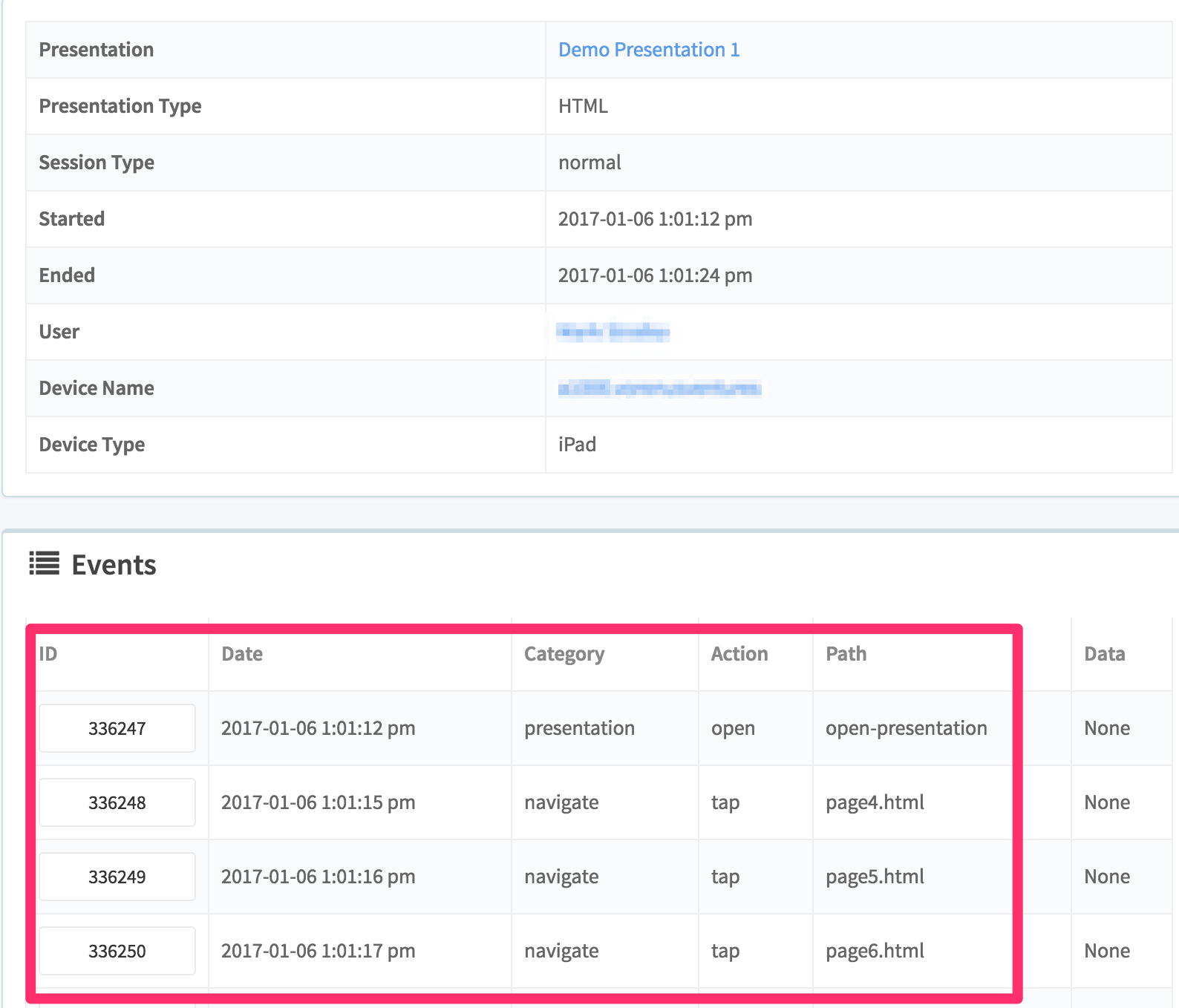
The logEvent
function takes 3 arguments:
Argument | Description |
---|---|
category | A high level grouping of what the user did. Examples: navigate , Video |
action | What the user did within that category: tap , open |
path (or uri) | The path or URL on which the event occurred: /products.html , /more-info.html , etc. |
// This example logs the user interacting with a video
mobilelocker.logEvent('Video', 'open', 'video-1.mp4');
mobilelocker.logEvent('Video', 'pause', 'video-1.mp4');
mobilelocker.logEvent('Video', 'fast-forward', 'video-1.mp4');
mobilelocker.logEvent('Video', 'close', 'video-1.mp4');
You can use the SDK to get logged events too.