You can insert a <form>
into an HTML presentation. Intercept the submit() event in JavaScript so the submitted data will be serialized to JSON and sent to the admin portal, where it can be exported to CSV or sent to another system using a webhook.
Example: Take attendance at a conference or event
Sample Code
Download this attendance-taking presentation here:
https://bitbucket.org/vorenusventures/kazaamax-attendance
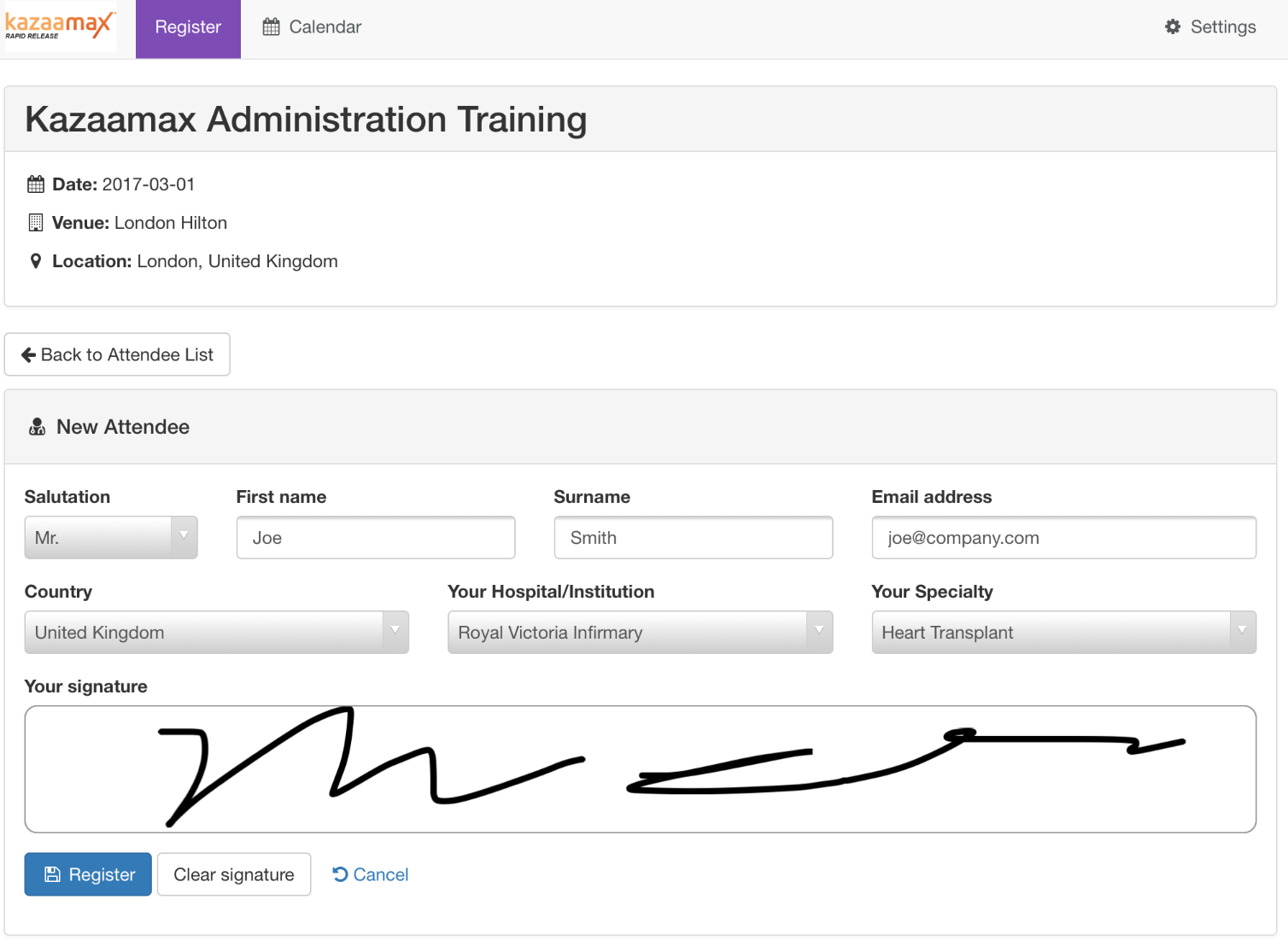
Example: Take attendance at a conference
View the submitted data in the admin portal
The data is logged as an event and viewable in the admin portal like this:
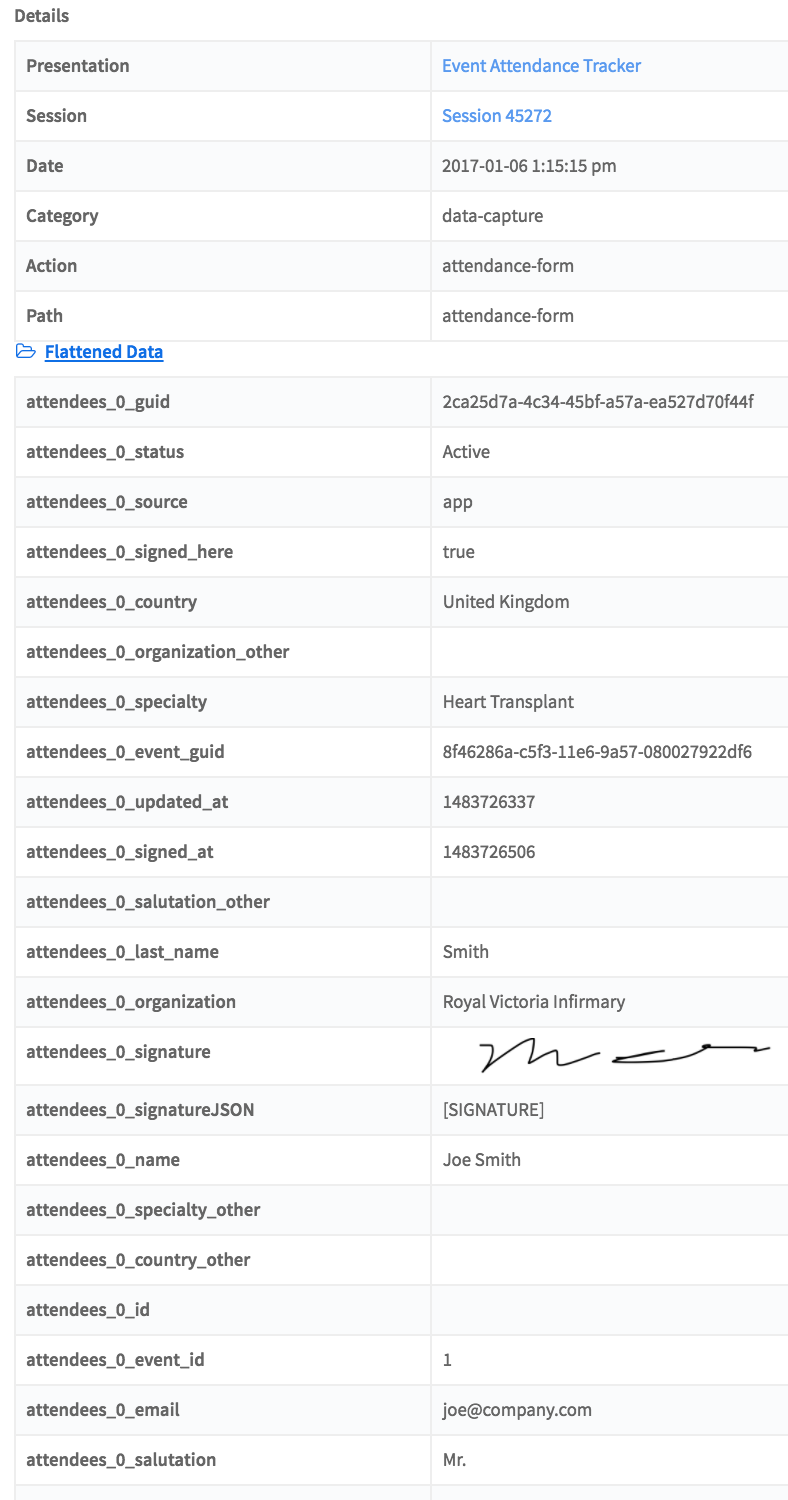
The submitted form data can be viewed in the admin portal.
Example Implementation
This example uses jQuery but the sample attendance app uses VueJS.
<form id="attendance">
<input type="text" name="first_name" value="Joe" />
<input type="text" name="last_name" value="Smith" />
<button type="submit">Submit</button>
</form>
$('#attendance').submit(function(event) {
event.preventDefault();
// https://api.jquery.com/serializeArray/
var tempData = $(this).serializeArray();
var formData = {};
// optional: convert the array to an object with the corresponding name-values
for (var i = 0; i < tempData.length; i++) {
formData[tempData[i].name] = tempData[i].value;
}
mobilelocker.submitForm('attendance-form', formData)
.then(data => {
alert('Thank you for registering');
});
return false;
});