Send a custom email directly from the JavaScript SDK
Instead of using the iOS Mail app to compose a custom email, you can also send an email directly through the SDK from JavaScript. You may want to do this if your company requires the email to have specific content, or users are not allowed to write a custom message.
Use Case
An HTML presentation may have many PDF files. A user may want to send one of these PDF files to a customer via email, and that email needs to be in a specific template.
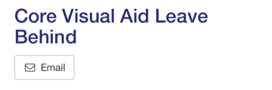
Tap on the Email button to open a form like this:
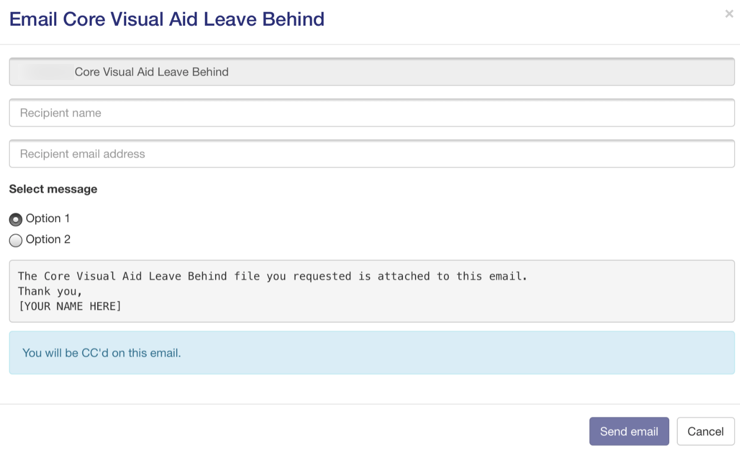
This example shows more advanced functionality to select from two messages.
Send the email with personalized content
In your HTML, build a form to capture the recipient's name and email address, and any other fields, as described in Capture a form in a presentation:
<form onsubmit="submitForm()">
<div class="form-group">
<label>Recipient Name</label>
<input type="text"
class="form-control"
id="recipient_name"
placeholder="Recipient name"/>
</div>
<div class="form-group">
<label>Recipient Email</label>
<input type="email"
class="form-control"
id="recipient_email"
placeholder="Recipient email"/>
</div>
<button type="submit"
class="btn btn-primary">
Submit
</button>
</form>
In your JavaScript, create a form object like this:
function initForm() {
return {
email: {
attachment: {
name: 'Core Visual Aid',
path: 'pdfs/Core_Visual_Aid.pdf', // Relative path to file from the root of the presentation
},
recipient: {
name: 'John Doe',
email: '[email protected]',
bcc_user: false, // IF true, the ML user will be BCC'd on the message.
cc_user: false, // IF TRUE, the ML user will be CC'd on the message.
},
subject: 'Company: Core Visual Aid',
body: '<p>The Core Visual Aid Leave Behind file you requested is attached to this email.</p>', // Use this if you want to personalize the body of the email.
template: 'templates/product_xyz.html', // Use this instead of "body" if you don't need to personalize the content of the email. It is the eelative path to file from the root of the presentation
}
}
}
In your submit handler, call the sendEmail
function:
/**
* Send an email to a recipient
* @param {string|object} to - Required - The recipient's email address or an object with "name" and "email" properties
* @param {string} subject - Required - The subject line
* @param {string|null} body optional - The HTML body of the email.
* @param {string|null} attachment - optional attachment path, relative to the root of the presentation (no slash prefix).
* @param {string|null} template - optional path for the HTML template, relative to the root of the presentation (no slash prefix). If not provided, the default Mobile Locker template will be used.
* @param {object|null} formData - optional object with additional data
* @returns {Promise}
*/
function sendEmail(to, subject, body = null, attachment = null, template = null, formData = null)
Or do something like this:
function submitForm(event) {
event.preventDefault();
const form = initForm();
// Use vanilla Javascript, Vue, jQuery, or another framework to update the form object's properties with the values from your user's input form
form.email.recipient.name = document.getElementById('recipient_name');
form.email.recipient.email = document.getElementById('recipient_email');
form.email.body = personlizeBody(form); // Your custom function to personalize the content of the email.
form.email.template = null; // If you're not using a template
mobilelocker.submitForm('email_form', form)
.then(result => {
alert('Success');
// handle the success response
})
.catch(err => {
// handle the error
});
}
Your personalizeBody()
function can generate HTML, with whatever combinations of images, styles, and text. Images and other static assets can be referenced as Public Files.
/**
* Send an email to a recipient
* @param {string|object} to - Required - The recipient's email address or an object with "name" and "email" properties
* @param {string} subject - Required - The subject line
* @param {string|null} body optional - The HTML body of the email.
* @param {string|null} attachment - optional attachment path, relative to the root of the presentation (no slash prefix).
* @param {string|null} template - optional path for the HTML template, relative to the root of the presentation (no slash prefix). If not provided, the default Mobile Locker template will be used.
* @param {object|null} formData - optional object with additional data
* @returns {Promise}
*/
function sendEmail(to, subject, body = null, attachment = null, template = null, formData = null)
The data is submitted to Mobile Locker as a standard form event. The special email_form form name parameter will cause the Mobile Locker system to process this event differently, sending it as an email to your recipient.
The recipient will receive the PDF attached to the email.
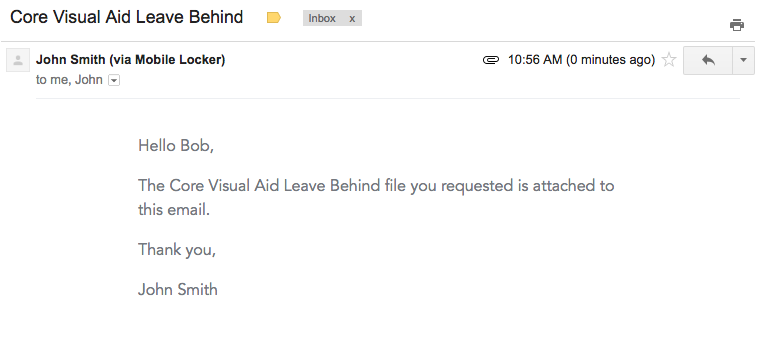
Your custom email can be more visually impressive than this example, but keep in mind that "simple" HTML emails are more likely to get past spam filters.
Test the deliverability of your custom emails
You should test your emails through a service like Mail Tester to increase the likelihood of the email getting past a recipient's corporate spam filter. This is especially important if you are a pharmaceutical company because spam filters are more likely to block emails about pharmaceutical products than other types of outreach emails.